The Official RDrama Computer Science Reading Group
My dear !codecels, hello and welcome to the first meeting of RDrama's Computer Science Reading Group! Here's the idea - we (read: I) pick a computer science textbook, then post a list of sections and exercises from that textbook each week. In the thread, feel free ask questions, post solutions, and bully people for asking stupid questions or posting stupid solutions. If you don't want to read along, I'll post the complete exercises in the OP, so you can solve them without needing to read the book.
SICP
The book I'm starting with is 'the Structure and Interpretation of Computer Programs' (abbreviated SICP). It's a software engineering textbook written by Gerald Jay Sussman and Hal Abelson from MIT. The book builds programming from the ground up: starting with a very simple dialect of Scheme and growing it into a language with lazy evaluation, object-orientation and a self-hosting compiler. It's a fun book: the exercises are hands-on and interesting, the writing is informative without being droll, and both the book itself and a corresponding lecture series (complete with a 80s synth rendition of 'Also Sprach Zarathustra') are available for free online.
Languages
The book uses (a small subset of) Scheme as its primary language, but feel free to try using a different language. The book's dialect of scheme is available through Racket, but most lisps will work with only minor changes. Other dynamically-typed, garbage-collected languages with higher-order functions will also not require much hacking: there is an edition written in JavaScript , as well as a partial adaptation to python
. High-level, statically typed languages might also work: Java/Kotlin/C#
seem doable, but I don't know those languages well. Strongly typed languages like Haskell will require some real hacks, and I'd avoid doing it in C, C++ or Rust.
Exercises
The book is split into five chapters:
- Building Abstractions with Procedures
- Building Abstractions with Data
- Modularity, Objects and State
- Metalinguistic Abstraction
- Computing with Register Machines
This week, I'll be posting exercises from the first chapter. The chapter is pretty easy for those familiar with programming already, so I just want to get it out of the way. Here are the selected exercises:
Exercise 1.8
Newton's method for cube roots is based on the fact that if
y
is an approximation to the cube root ofx
, then a better approximation is given by the value(x/y² + 2y) / 3
. Use this formula to implement a cube-root procedure which is wrong by at most0.01
.
Exercise 1.12
The following pattern of numbers is called Pascal's Triangle.
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
...
The numbers at the edge of the triangle are all 1, and each number inside the triangle is the sum of the two numbers above it. Write a procedure that computes elements of Pascal's triangle.
Exercise 1.18
Devise a procedure generates an iterative process for multiplying two integers in terms of adding, doubling, and halving and uses a logarithmic number of steps.
Exercise 1.31
Write a procedure called
product
that returns the product of the values of a function at points over a given range (product(l, r,step,f) = f(l) * f(l+step) * f(l + 2 * step) * ... * f(r)
). Show how to definefactorial
in terms ofproduct
. Also use product to compute approximations to using the formulaπ/4 = (2 * 4 * 4 * 6 * 6 * 8 ...) / (3 * 3 * 5 * 5 * 7 * 7 ...)
Exercise 1.43
If
f
is a numerical function andn
is a positive integer, then we can form then
th repeated application off
, which is defined to be the function whose value atx
isf(f(...(f(x))...))
. For example, iff
is the functionx → x + 1
, then then
th repeated application off
is the functionx → x + n
. Iff
is the operation of squaring a number, then then
th repeated application off
is the function that raises its argument to the2 * n
th power. Write a procedure that takes as inputs a procedure that computesf
and a positive integern
and returns the procedure that computes then
th repeated application of f. Your procedure should be able to be used as follows:repeated(square,2)(5) = 625
Have fun!
Jump in the discussion.
No email address required.
!commenters - it seems like people didn't really care about this thread, so I don't think I'm going to continue it. I had hoped it'd be like the advent of code threads on /g/, but it seems like there aren't enough people here to make the thread more than me shouting into the void.
Jump in the discussion.
No email address required.
advent of code is masturbatory and only attracts the worst people.
Jump in the discussion.
No email address required.
Where do you think we are?
Jump in the discussion.
No email address required.
post blahaj and socks
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
Here are my answers in Racket. Last two questions were the toughest. Might be worth to split future chapters into 2-3 weeks.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
SICP for GNU Info
https://pomf2.lain.la/f/h2scvw9t.info
Jump in the discussion.
No email address required.
More options
Context
Why arent you converting the SICP exercises to a different language
Jump in the discussion.
No email address required.
Do you mean the literal 40 bytes of code that was in prefix notation? Fine - I'll add some JS notation for the code-monkeys.
Jump in the discussion.
No email address required.
I remember one of you mentioned some.book and how it was great except it used some language that was disliked.
Jump in the discussion.
No email address required.
Literally every language has people who dislike it. I don't know if "some book" referred to SICP and "some language" referred to scheme, but if that's the case, then it already has been translated to JavaScript and Python, like I said in the OP.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
I'm a mathlet, my original plan was reading Knuth's Concrete Mathematics and then "Coding the Matrix" for linear algebra before SICP but I'm not doing that shit
I just want to coode 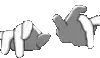
Should I just jump right into SICP?
Jump in the discussion.
No email address required.
Why?
Sure - SICP is as good an intro book as any. It is a bit abstract and won't teach you a popular language, but it will definitely teach you how to go about writing code.
Jump in the discussion.
No email address required.
Idk its just what was recommended to me, concrete mathematics for a base and coding the matrix for linear algebra but I've been out of formal education too long and it's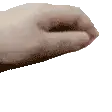

I already know how2coode I just want to better understand CS
Jump in the discussion.
No email address required.
Honestly just make projects that vaguely touch what you want to learn. Do you want to learn autismo optimization? Learn C/C++/Rust and learn about how memory allocation works, wanna make a basic telnet/SSH client as an intro to networking? Java/C#/Kotlin has a library for that. Do you want better stackoverflow answers? Find a moderately obscure language (like any Lisp) and watch all those
wash away.
Literally just make projects, then find books and read relevant parts of them whenever you hit a wall. That's what I'd go for anyway, since the entire point of understanding CS is to write better programs, if you're not gonna write programs, idk what to say. I'll also add that most programming books tend to be a lot slower than necessary in my experience.
Jump in the discussion.
No email address required.
More options
Context
CS is a big field, so "understanding CS" is a hard thing to do. If you want to learn more about programming languages and software engineering, SICP is a good start. Otherwise, there are good books on Compilers, Operating Systems, Graphics Programming, Theory, etc.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
Thanks for hosting this. I have now got around to reading the first chapter. If you decide to continue this series, it is likely that I will always need more time than a week.
I understand now why the book is so beloved. The first chapter starts of very strongly. The quote by John Locke particularly surprised me. I always thought that this is a very computer sciency way to look at things, but it appears that we just got inspired by a philosophy much older than computers.
The wizard analogy is also pure kino and I now finally understand the memes I'm spamming.
Not gonna reproduce the exercises here, I just did a few of them to practice the syntax. I definitely see the elegance of it, thoughbeit I don't know yet whether I find parens-soup aesthetically pleasing.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
What a ridiculous little culture.
Jump in the discussion.
No email address required.
More options
Context
Do introduction to computing by Yale patt. Let's do some assembly for the lc3
Read what I wrote above. Now picture in your head that I put a /s at the end. Good job sweaty!
Jump in the discussion.
No email address required.
I haven't read that book: it seems similar to the Nand-to-tetris book, but less fun
Jump in the discussion.
No email address required.
It's extremely informative though. Probably learned more in that class and digital logic design than any other classes in school
Read what I wrote above. Now picture in your head that I put a /s at the end. Good job sweaty!
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
Motherlover, I had a .edu email before .com TLDs existed. All y'all AOLn00bs think you're fresh; you're just trite.
Snapshots:
https://i.kym-cdn.com/photos/images/original/001/584/970/18c.png:
ghostarchive.org
archive.org
archive.ph (click to archive)
the book itself:
ghostarchive.org
archive.org
archive.ph (click to archive)
corresponding lecture series:
ghostarchive.org
archive.org
archive.ph (click to archive)
available through Racket:
ghostarchive.org
archive.org
archive.ph (click to archive)
an edition written in JavaScript:
ghostarchive.org
archive.org
archive.ph (click to archive)
partial adaptation to python:
ghostarchive.org
archive.org
archive.ph (click to archive)
Jump in the discussion.
No email address required.
More options
Context