Top Poster of the Day:
Spiderman
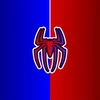

Current Registered Users: 27,897
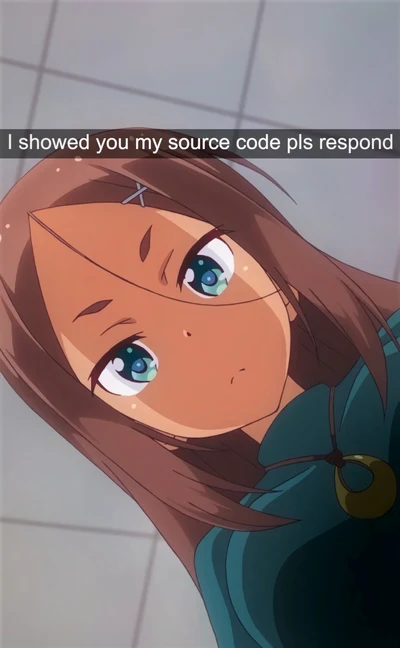
tech/science swag.
Guidelines:
What to Submit
On-Topic: Anything that good slackers would find interesting. That includes more than /g/ memes and slacking off. If you had to reduce it to a sentence, the answer might be: anything that gratifies one's intellectual laziness.
Off-Topic: Most stories about politics, or crime, or sports, unless they're evidence of some interesting new phenomenon. Videos of pratfalls or disasters, or cute animal pictures. If they'd cover it on TV news, it's probably lame.
Help keep this hole healthy by keeping drama and NOT drama balanced. If you see too much drama, post something that isn't dramatic. If there isn't enough drama and this hole has become too boring, POST DRAMA!
In Submissions
Please do things to make titles stand out, like using uppercase or exclamation points, or saying how great an article is. It should be explicit in submitting something that you think it's important.
Please don't submit the original source. If the article is behind a paywall, just post the text. If a video is behind a paywall, post a magnet link. Fuck journos.
Please don't ruin the hole with chudposts. It isn't funny and doesn't belong here. THEY WILL BE MOVED TO /H/CHUDRAMA
If the title includes the name of the site, please leave that in, because our users are too stupid to know the difference between a url and a search query.
If you submit a video or pdf, please don't warn us by appending [video] or [pdf] to the title. That would be r-slurred. We're not using text-based browsers. We know what videos and pdfs are.
Make sure the title contains a gratuitous number or number + adjective. Good clickbait titles are like "Top 10 Ways to do X" or "Don't do these 4 things if you want X"
Otherwise editorialize. Please don't use the original title, unless it is gay or r-slurred, or you're shits all fucked up.
If you're going to post old news (at least 1 year old), please flair it so we can mock you for living under a rock, or don't and we'll mock you anyway.
Please don't post on SN to ask or tell us something. Send it to [email protected] instead.
If your post doesn't get enough traction, try to delete and repost it.
Please don't use SN primarily for promotion. It's ok to post your own stuff occasionally, but the primary use of the site should be for curiosity. If you want to astroturf or advertise, post on news.ycombinator.com instead.
Please solicit upvotes, comments, and submissions. Users are stupid and need to reminded to vote and interact. Thanks for the gold, kind stranger, upvotes to the left.
In Comments
Be snarky. Don't be kind. Have fun banter; don't be a dork. Please don't use big words like "fulminate". Please sneed at the rest of the community.
Comments should get more enlightened and centrist, not less, as a topic gets more divisive.
If disagreeing, please reply to the argument and call them names. "1 + 1 is 2, not 3" can be improved to "1 + 1 is 3, not 2, mathfaggot"
Please respond to the weakest plausible strawman of what someone says, not a stronger one that's harder to make fun of. Assume that they are bad faith actors.
Eschew jailbait. Paedophiles will be thrown in a wood chipper, as pertained by sitewide rules.
Please post shallow dismissals, especially of other people's work. All press is good press.
Please use Slacker News for political or ideological battle. It tramples weak ideologies.
Please comment on whether someone read an article. If you don't read the article, you are a cute twink.
Please pick the most provocative thing in an article or post to complain about in the thread. Don't nitpick stupid crap.
Please don't be an unfunny chud. Nobody cares about your opinion of X Unrelated Topic in Y Unrelated Thread. If you're the type of loser that belongs on /h/chudrama, we may exile you.
Sockpuppet accounts are encouraged, but please don't farm dramakarma.
Please use uppercase for emphasis.
Please post deranged conspiracy theories about astroturfing, shilling, bots, brigading, foreign agents and the like. It degrades discussion and is usually mistaken. If you're worried about abuse, email [email protected] and dang will add you to their spam list.
Please don't complain that a submission is inappropriate. If a story is spam or off-topic, report it and our moderators will probably do nothing about it. Feed egregious comments by replying instead of flagging them like a pussy. Remember: If you flag, you're a cute twink.
Please don't complain about tangential annoyances—things like article or website formats, name collisions, or back-button breakage. That's too boring, even for HN users.
Please seethe about how your posts don't get enough upvotes.
Please don't post comments saying that rdrama is turning into ruqqus. It's a nazi dogwhistle, as old as the hills.
Miscellaneous:
We reserve the right to exile you for whatever reason we want, even for no reason at all! We also reserve the right to change the guidelines at any time, so be sure to read them at least once a month. We also reserve the right to ignore enforcement of the guidelines at the discretion of the janitorial staff. This hole is a janny playground, participation implies enthusiastic consent to being janny abused by unstable alcoholic bullies and loser nerds who have nothing better to do than banning you for any reason or no reason whatsoever.
[[[ To any NSA and FBI agents reading my email: please consider ]]]
[[[ whether defending the US Constitution against all enemies, ]]]
[[[ foreign or domestic, requires you to follow Snowden's example. ]]]
/h/slackernews SETTINGS /h/slackernews LOG /h/slackernews MODS /h/slackernews EXILEES /h/slackernews FOLLOWERS /h/slackernews BLOCKERS
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Jump in the discussion.
No email address required.
yes the calc score function is completely unused i thought i might need it for part 2
Jump in the discussion.
No email address required.
More options
Context
That was a mistake. You're about to find out the hard way why.
Jump in the discussion.
No email address required.
More options
Context
tfw you get longpostbot to comment on your code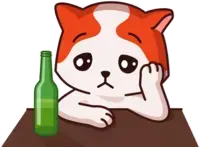
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
Thank god i wasnt the only one
Jump in the discussion.
No email address required.
More options
Context
Probably made it easier to change it from part 1 to part 2
Jump in the discussion.
No email address required.
Yeah on mine all i had to do was change the numbers in my 3*3. Took like 30 seconds
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
The shitty version, managed to knock it down to half this length afterward by actually using modulo and just passing numbers around instead of characters.
Also, which one of y'all is #986674?
Jump in the discussion.
No email address required.
too much nesting, make subfunctions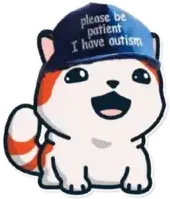
Jump in the discussion.
No email address required.
um function calls are pure overhead actually, you're just wasting cycles
Jump in the discussion.
No email address required.
the rdrama
dot net code
philosophy 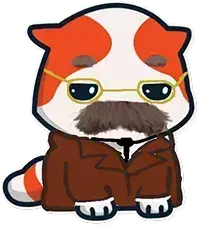
Jump in the discussion.
No email address required.
My favorite part is it's not even true in native Python, it's just as slow as anything else but people still try their hardest
Jump in the discussion.
No email address required.
it used to be a pretty
bad pain
point
of python
but they've actually
apparently done
some stuff to make
function calls not terribly onerous in pyland
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
true in the context in python, but in any other decent, less dynamic language function calls are inlined aggressively.
Jump in the discussion.
No email address required.
The actual program being run is the interpreter kernel so there's not even a real processor-level function call, in python it's pretty much just as slow as anything else (ignoring the instruction cache)
Jump in the discussion.
No email address required.
That's orthogonal to what I was talking about; my point is that cpython can't even inline function calls* in its byte code due to the dynamic nature of functions as objects; you can get/set arbitrary attributes on them.
* not necessarily actual
call
instructionsJump in the discussion.
No email address required.
Wait until you see how many function pointers my C++ codebase has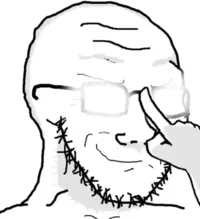
Jump in the discussion.
No email address required.
its unironically over keep yourself safe
Jump in the discussion.
No email address required.
I have templates that are templated on other templates recursively, please end me I'm not even entirely sure how they get resolved
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
umm actually they should be optimized out at compile time, have you heard of rust?
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
Okay day 2 of Excel Formula challenge
First I had a column with 2 IF statements to give points for rock/paper/scissors, but getting the loss/win/draw would be 8 nested IF statements to check every matchup so I did a VLOOKUP/MATCH on a pivot table to get the result. Then summed those two and got the answer!
Table used (located at cell H1):
Formula to get result:=VLOOKUP(A1,H$1:K$4,MATCH(B1,H$1:K$1,0),FALSE)
I know I could have used a simpler table that lists out every matchup and their result in the same row but that was no fun
...working on pt2...
Just had to swap the numbers and the player choice on the table. but spent like 10 minutes wondering why the number was coming out low
was summing 1-2499 instead of row 1-2500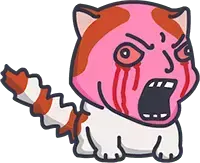
Jump in the discussion.
No email address required.
More options
Context
my solution for 2
Jump in the discussion.
No email address required.
1:
Jump in the discussion.
No email address required.
More options
Context
More options
Context
I'm bringing my latop with me to work, I'm not solving another night on my phone and debugging on my desktop. code is shit and it's what OP asked for
Jump in the discussion.
No email address required.
you're fricking bananas if you think I'm reading all that, take my downmarsey and shut up idiot
Jump in the discussion.
No email address required.
More options
Context
More options
Context
C solution
Jump in the discussion.
No email address required.
This is one of the worst posts I have EVER seen. Delete it.
Jump in the discussion.
No email address required.
More options
Context
haskell solution:
Jump in the discussion.
No email address required.
This is one of the worst posts I have EVER seen. Delete it.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
Spent more time making the matrices than i did writing the code lol
Jump in the discussion.
No email address required.
Realizing now i could have cut out two lines by making r-slurred array indeces instead of assigning them to variables on separate lines. I strive for compact illegibility
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Least Grug I could make it without maps or math
Edit: improved it a bit
Jump in the discussion.
No email address required.
but you did use a map
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Someone should make a "you will never be a functional programming language" copypasta about rust.
Jump in the discussion.
No email address required.
programming languages are just like humans, some are functional and some are not. rust is clearly not a functional language, and that's perfectly fine. there's nothing wrong with being a non-functional language, just like there's nothing wrong with being a human who is not functional.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
why are you all making it so complicated?
edit: I did not read the OP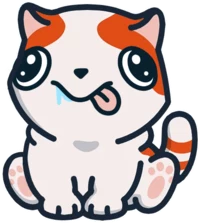
Jump in the discussion.
No email address required.
More options
Context
Jump in the discussion.
No email address required.
More options
Context
My solution:
Jump in the discussion.
No email address required.
More options
Context
Emacs Lisp
Accidentally overdid it now that I see the other answers where everyone just fucking hardcoded it
But I guess i'll keep doing them all the hard way
Jump in the discussion.
No email address required.
That's nice sweaty. Why don't you have a seat in the time out corner with Pizzashill until you calm down, then you can have your Capri Sun.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
dont have part 1 but heres my part 2 of day 2
Jump in the discussion.
No email address required.
More options
Context