TempleOS, that esoteric operating system developed by a schizophrenic guy who loved saying the n word. You've probably heard of it and occasionally get reminded of its existence when you see and
. It's probably of no value and only /g/ schizos use it to get called a gigachad, don't they? Who else could _possibly_ use it? Well, here's one that went as far as to porting it to ring 3 and blogposts about it on rDrama of all sites because hes too lazy to even setup a https://github.io blog.
Ok, interested? So basically I effectively made TempleOS an app that can be launched from Linux/Windows/FreeBSD and be used as either an interpreter that could be run from the command line, or as just a vm-esque orthodox TempleOS GUI that you could use just like TempleOS in a VM, just faster (no hardware virtualization overhead) and more integrated with the host. It doesn't have Ring 0 routines like InU8 so it doesn't have that "poking ports and seeing what happens" C64core fun though, so keep that in mind.
It also has a bunch of community software written for TempleOS like CrunkLord420's BlazeItFgt1/2, a DOOM port, and a CP/M emulator written in HolyC. Try them out! There's also networking implemented with C FFI and an IRC server+client and a wiki server in the repository that uses it, if you're concerned whether Terry would think it's orthodox, it's totally ok. You could read more about why in the repo readme.
Here's a simple showcase, this would show you the gist/rationale of making this software.
So let me go on a journey of longposting about how I ported TempleOS to Ring 3.
Step 1. The kernel
There's a _lot_ of stuff in TempleOS that's ring 0 only. No wonder, since Terry always was adamant about being able to easily fuck with the hardware in a modern OS. But on the other hand, this makes porting TempleOS a _lot_ easier. Since the whole operating system is ring-0 only and is a unikernel, every processes share the same address space and that means you could run all the kernel code confined in a single process running on top of another opreating system and have no problems with context switching and system calls, they're all just going to be internal function calls inside the process itself.
Now with this idea, what could we do? We have to study the anatomy of the kernel to be able to port it, of course.
This blog explains it in much more detail, but here's the gist:
You have a bunch of code, but it's incomplete. There's a "patch table" that has the real relocation addresses for the CALL addr
instructions, and you fill them in, this sounds easy enough. Plus, TempleOS already has the kernel loader written. We're sneeding that.
Here's some of the code, but it's irrelevant. Let's move on.
But wait, it shouldn't be this easy. How does TempleOS layout its memory? Let's check the TempleOS docs. Did I mention TempleOS is far more well documented than any other open source software in the world?
Step 1.5. The memory and the poop toad in the secret sauce
That's amazing! Let me quote a few important parts:
So what does this mean? We need RWX (read+write+execute) memory pages mapped in the process' lower 2 gigabytes, and normal memory maps anywhere else. This is great because we don't have to care too much about where we should place memory. Plus, memory is "identity-mapped" (host memory would directly mirror TempleOS' internal memory addresses) so no worries about address translation.
Here's the code only for the POSIX part of the virtual page allocator because it's more elegant. It's a simple bump allocator with mmap. Works on all Unixes except OpenBSD because they won't allow RWX unless you do weird stuff like placing the binary on a special filesystem with special linker flags because of security theater measures. Theo, nobody uses your garbage.
End step 1.5
We're going to have to strip out all the stuff that does ultra low-level boot/realmode stuff. This was a really long tedious thing to do and I'm not enumerating everything I removed. Ok, so what's next?
Step 2. Getting it to compile
How are we going to get this to compile in the first place? Well, turns out the HolyC compiler can AOT compile too alongside the JIT compilation mode it's known for.
So we write a file that just includes all the stuff to make a kernel binary. This part is very short but we're in for a ride, bear with me.
Step 3. i can haz ABI plz?
How do we call HolyC code from C, and vice versa? Intuitively you should know that this is a requirement. Let's check the docs again.
Ok, cool. This means that
1. TempleOS ABI is very simple
All arguments are passed on the stack, Variadics are also very easy. Take a look at this disassembly:
PUSH 0043BBED pushes the address for the string "Hello rDrama" on the stack, PUSH 17 pushes 0x17(23), the second argument to the stack. PUSH 02 means there are two variadic arguments, and you could access it from the function as argc. argv points the start of the two variadic arguments we pushed on the stack. Much simpler than the varargs mess you see in C, right?
Here's an additional diagram for a C FFI'd function that the HolyC side calls variadically.
2. FS/GS is used for thread-local storage - the current process in use and the current CPU structure the core has.
this is VERY important. Don't miss this if you're actually reading this stuff. All HolyC code is a coroutine, you call Yield() explicitly to switch to the next task. There is no preemptive multitasking/multicore involved. Everything is manual. Fs() points to the current task which gives HolyC code a OOPesque
this
pointer that you pass in routines involving processes, and you can use them for any process - be it yours or another task and easily play around with them.
So how do we implement Fs and Gs? Simple, thread-local variables in C. We'll come back to C very soon
Sorry if you were disappointed in the implementation, lol
3. Saved registers
You save RBP, RSI, R10-R15. That's the only requirement for calling into/being called from HolyC.
Here's how I implemented HolyC->C FFI. Save all the HolyC registers, and have placeholders for CALL instructions that you fill in later, kinda like the TempleOS kernel itself. We move the HolyC arguments' starting address to the first argument in the host OS' calling convention, so an FFI'd C function looks like this:
How do we implement C->HolyC FFI btw? Well, it's vice versa, but this time we push all the host OS' register/stack arguments on the HolyC stack.
I wrote a complete schizo asm generator for this that I assemble & link into the loader.
Step 4. Seth, bearer of multicores
The core task in TempleOS in called Seth, from a bible reference. This turned out to be relatively easy, after we load the kernel and extract the entry points from it, we simply execute all of them in the core with the FFI stuff we just wrote above.
This shouldn't be this easy. What are the caveats? And what are those signal handlers?
Well, we need to add Ctrl-Alt-C support, which is basically CTRL^C in TempleOS. HolyC, as mentioned above, doesn't have preemption, so an infinite loop without a yield will freeze the whole system. How do we break out of this?
We use signal handlers in Unix for this. Basically we use the idea that the operating system will force execution to jump to the signal handler when a signal is raised.
On Windows, it's a bit more sassy. Windows has the ability to suspend threads remotely and get a dump of the registers, and resume it.
Step 5. User Input/Output
I use SDL for the GUI input/output and sound (TempleOS has BIOS PC speaker mono beeps for sound, it's very simple), and libisocline for CLI input. I'm not going into super specifics because it's boring as fricc.
Step 6. Filesystem integration
TempleOS uses drive letters like C: and D:. We need to translate these ondemand for the kernel to access files.
This is the heart of the virtual filesystem. It's quite simple. We just strcpy a directory name into a thread-local variable, and basically have an alphabet radix table.
I just wanted to show you guys this part. It's a file truncation routine & its super lit, we can throw HolyC exceptions from C because throw() is a function in HolyC.
Small "logic switch" thing I did for the poor man's Rust match
, thought it was neat. (Writing EXODUS in Rust would not be fun. Unsafe Rust gets ugly quick, and I've tried writing some unlike the /g/ chuds)
Step 7. Debugging
Now, we've almost reached the end. At this point, you can run stuff just fine with our TempleOS port. But, how do we debug HolyC code?
TempleOS has a very, _very_ primitive debugger. I thought this was _too_ primitive for my taste, so I gave it a modern spin:
Looks much better, and more orthodox in a way.
I just dump all the registers when I catch a SIGSEGV or anything else that indicates a bug and send it to the HolyC side.
Step 7.5. Backtrace
How do we get the backtrace of the HolyC functions? Fear not, because the kernel calls a routine that adds all the HolyC symbols to the C side's hash table in the boot step. Now that we have all the symbols what do we do?
Here's the anatomy of an x86_64 function if you don't know:
RBP(stack BASE pointer) points to the previous RSP(stack pointer) of the callee of the function, and RBP+8 points to the return address, which means where the function, after returning, will resume its execution at. Now with this knowledge, how do we implement a backtrace?
We keep drilling down the call stack and grabbing RBP+8's so we know which functions called the problematic function, and find the address offsets in the symbol table with a linear search.
end step 7.5
Congratulations, this is the end. This probably covers more than your average university CS semester. My stupid ass can't articulate this in a juicier way sorry.
Trivia
Terry never used dynamic arrays (vectors). He always used circular doubly-linked lists because they're much more elegant to use in C. Really, there's no realloc too. (
<(data locality be damned) its actually not that bad.)
HolyC typecasts are postfix, this is to enable stuff like
HashFind(...)(CHashSrcSym*)->member
which makes pseudo-OOP much cleaner. HolyC has primitive data inheritance. (this one is code to retrieve a symbol from a hash table, HashFind returns aCHash*
butCHashSrcSym
inherits fromCHash
)"abcd %d\n",2;
is shorthand for printfHolyC has "subswitches", like destructors and constructors for a range of cases.
Cool, huh? It's very useful for parsers.
I mentioned this eariler but let me reiterate: All HolyC code is a coroutine. You explicitly yield to Seth, the scheduler, for context switching between tasks. This is how cooperative multitasking should be done, but only Go does it properly, but even then they're not the real deal by mixing threads with coroutines.
IsBadReadPtr() on Windows friccin sucks. Use VirtualQuery. You can do the same thing in Unixes with msync(2) (yeah wtf. it's originally for flushing mmapped files but hey, it works)
There's a ton I left out for the sake of brevity but I invite you to read the codebase if you want to dig deeper.
Big thanks to nrootconauto who introduced me and led me through a lot of Terry's code and helped me with some HolyC quirks. He has his own website that's hosted on TempleOS and it's lit. Check it out.
There's probably more but I think this is enough. Thanks for reading this, make sure to leave a star on my repo if you can
Jump in the discussion.
No email address required.
glowies don't want you too know this, but TempleOS is free. You can hear God's own voice in you're Unix system. @JimieWhales hear God in ring 3 all the time. She tells @JimieWhales that black trans lives matter.
Jump in the discussion.
No email address required.
!schizos
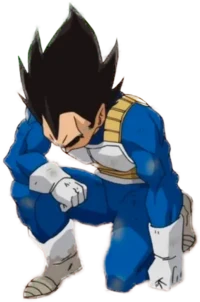
Jump in the discussion.
No email address required.
CIA AGENTS ARE AFRAID OF GD, THEY DON'T WANT THE PEOPLE TO BECOME MORE DIVINE BY CONNECTING THEMSELVES WITH GD. IT IS ALL THE FAULT OF THE GAY ILLUMNATI ORDER WHO WANTS TO ENSLAVE THE POPULATION AND FORCE THEM TO LIVE IN A POD AND EAT ZE BUGS. IT IS ALL TRUE I'VE DONE MY RESEARCH I'VE LOOKED IT UP
trans lives matter
Jump in the discussion.
No email address required.
More options
Context
Templeos is God's binary whisper
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
wtf this is too high effort for us
!codecels
Follower of Christ
Tech lover, IT Admin, heckin pupper lover and occasionally troll. I hold back feelings or opinions, right or wrong because I dislike conflict.
Jump in the discussion.
No email address required.
thanks friend, its kind of all over the place though. i should refine this into a proper blogpost sometime later (never)
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Holy shit you are literally the most vile person that I have ever had the displeasure of interacting with on the internet. You might just be my first real block, I hate you with all of my guts. Every time there is a post about a cute little animal on the front page you're always the #1 report about wanting to frick its brains out. I hate you. And when it's not an animal post you're just begging for unbans. Why don't you take your bans with pride like me and stop being a whining b-word?
Snapshots:
ghostarchive.org
archive.org
archive.ph (click to archive)
https://github.io:
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
This blog:
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
it's very simple:
ghostarchive.org
archive.org
archive.ph (click to archive)
ghostarchive.org
archive.org
archive.ph (click to archive)
Jump in the discussion.
No email address required.
More options
Context
this is incredible
if your writeup wasnt posted on this gay s*x website I would show it to all my coworkers, as it is I like my job too much to share your brilliance
Jump in the discussion.
No email address required.
thanks! i could post this on a real blog someday because the effort required to set up a real blog is too much for me
(instant death)
Jump in the discussion.
No email address required.
use substack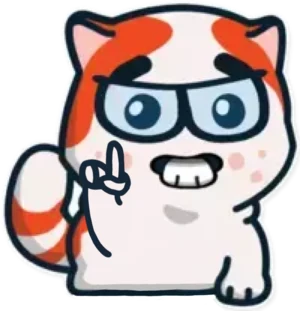
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
We will all use TempleOS in the future.
Jump in the discussion.
No email address required.
More options
Context
I'd suck your peepee for the effort but it's obviously too big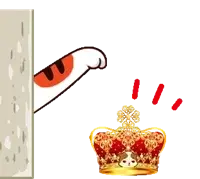
Jump in the discussion.
No email address required.
More options
Context
If only I knew how to code.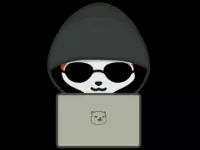
Good job though.
This is the official position of C-SPAN. If you have any questions or comments, please call and say something unhinged.
Jump in the discussion.
No email address required.
(thanks friend)>
it's not that hard to learn! just grab a project big enough for you to learn stuff instead of just grinding leetcode or whatever meme plebs follow. This was actually one of my first "real" projects that I've ever done, and i started when i had absolutely no idea what i was doing, and now I could confidently say i btfo most codecels
. (you can do it too)> 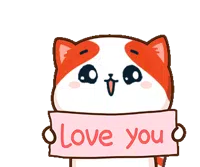
Jump in the discussion.
No email address required.
Funnily enough I actually asked my brother who's in undergrad for CS for a copy of his homework, and tried my best to race him. I didn't win, but I came close.
I really just copy and pasted until I met the requirements of CS 1100 lmao. It was tough but I had fun. With data becoming so important I'm struggling to determine if I double down in humanities or branch out into data/CS… it's tough.
This is the official position of C-SPAN. If you have any questions or comments, please call and say something unhinged.
Jump in the discussion.
No email address required.
At least learn how to script formula and automation in Excel to MOG your co-workers and look like a maverick wizard to your boss
!besties
Jump in the discussion.
No email address required.
That only works on kiddie datasets
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Digital humanities is a good grift.
Jump in the discussion.
No email address required.
Can you expand on that? I promise I won't chud you anymore.
I'm at a crossroads where I'm seeing my income potential hinge on either going into office or branching out, or taking a job with the government.
I wanna break that Sox figure barrier before I'm thirty lmao. But I also don't want to hate myself lmao.
This is the official position of C-SPAN. If you have any questions or comments, please call and say something unhinged.
Jump in the discussion.
No email address required.
I'm lowkey trying to bait you to chud me because I think it's funny. So uh, Die bipocs!
Digital humanities is pretty much like helping humanities profs that can't code. It was a dumb meme for a while, but now it's a real thing. If you want to stay in academia, it pays relatively well. You can break six figures easily in academic computing at an R1 university.
Jump in the discussion.
No email address required.
I thought it was pretty funny too, I only did it because I thought interpreting your irony literally was kinda funny.
And neat. I didn't know what that was per se, I've been out of academic circles for a while. I actually pushed back my entrance into a phd program because of my grandmother dying. It's been tough.
Honestly there's a lot of cool things people could do in the public/private partnership sphere that would be moneymakers, but most people in 3P spaces cannot code a lick.
Thanks for the explanation friend :)
This is the official position of C-SPAN. If you have any questions or comments, please call and say something unhinged.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
You're angering God by running TempleOS in userland.
I'm not even kidding. Terry Davis was clear that the display resolution was handed down from on-high, and you're messing with that mandate by putting TempleOS into userland.
Jump in the discussion.
No email address required.
the whole thing runs on 640x480 resolution, what are you talking about? it's the same way it'd be run on a VM.
Jump in the discussion.
No email address required.
You anger Him with your virtual machines.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
What if, instead, you had spent that effort making something that would improve anyone's life in any way?
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Yeah random
registered today to show this dead cat site xis epic meme project
I believe you
Jump in the discussion.
No email address required.
yes exactly
@A can confirm
Jump in the discussion.
No email address required.
Post picture of HRT bottle with a timestamp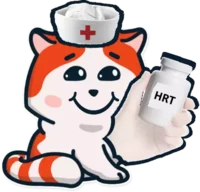
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
Dude
I didn't read all of this but will later. Are you going to try implementing networking?
Jump in the discussion.
No email address required.
oh i already did with the ffi interface thanks for reminding me! ill edit the post. there's a wiki and IRC server in the repo written in HolyC and they run just fine.
Jump in the discussion.
No email address required.
Lmfao
That's amazing
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
Very cool project.
If I understood correctly, you have basically replaced the Temple OS's syscalls that depend on hardware with C functions that emulate them. You load the TempleOS's kernel into a process address space in the lower 2GBs, spawn a few POSIX threads where each one simulates 1 CPU core, patch the symbol table with your own functions that do FFI into C code, and have the threads jump into the kernel's code.
3 questions:
How do you handle MMIO? Or does TempleOs not use any?
You force a "yield" in a process when you press ctrl-c, but wouldn't that risk breaking something due to race conditions? Or is that the same behavior also on TempleOS and you're expected to write software knowing it might context switch before a yield too?
I don't get why you need to manually save and restore the fs/gs registers. I would expect the TempleOS's kernel code to do all of that, and when one of your C functions end up getting called you have the running's task registers available there. What's thrashing them? Or are you just saving and restoring them at the entry and exit of the C function for the host's OS usage?
Jump in the discussion.
No email address required.
there's no memory-mapped IO because all the hardware is emulated with FFI shims. Of course there's memory-mapped IO for VGA and stuff in real TempleOS, that's what makes graphics programming a challenge in real TempleOS too. You need to write between the VGA blanks.
It's per-core.
FS/GS is used by the host operating system for thread-local storage already. It's gonna mess stuff up. Also TempleOS uses wrmsr to set the fs/gs base and im pretty sure thats kernel-priveliged stuff
Jump in the discussion.
No email address required.
So you can't run software written for TempleOS without changing its source a little bit. Still cool though.
Alright.
I am a bit confused by the execution model then. Once you let the TempleOS kernel run and it wants to context switch it's going to do try and run that instruction, so what's "connecting" the FS/GS variables you have defined to let TempleOS use those instead of the real registers? Do you have your own scheduler which replaces the TempleOS's one?
Jump in the discussion.
No email address required.
How? TempleOS doesnt just rawdog memory mapped ports all the time. That would be horrible. TempleOS has a bunch of routines you can use to interact with the hardware and most 3rd party programs for TempleOS are games anyway, which use DolDoc graphics routines. Your questions could be answered by just booting up TempleOS and looking around and reading the code, go do it.
What? the compiler is modified to call into C FFI instead of use the registers directly for them, since Fs/Gs are builtin functions that expand to
MOV RAX,FS:[RAX]
in stock TempleOS so we could edit that. Look at T/Compiler/BACKFA.HC's ICFs()/ICGs()Jump in the discussion.
No email address required.
Ah, alright. If user programs in TempleOS should not use direct hardware access then I don't get why Terry decided to run everything in ring 0
Ok, I missed the part where you modified the compiler to do that. That explains it
You're right, but I also won't do that
Jump in the discussion.
No email address required.
They can but do you never write functions for specific tasks? Do you just write 0s and 1s for everything? Probably not. Even in ring 0 you would want some automation. Terry wrote simple routines for manipulating the hardware that other routines use and I target them and replace them with FFI. Also I explained in OP that you wouldnt be able to poke around memory mapped ports and stuff in my userspace port. Most third party software for TOS dont really manually poke in hardware because TempleOS' standard library routines already have what they need, unless they're writing drivers for network cards or something.
Jump in the discussion.
No email address required.
Tell me more about how you don't code by telling me more about how you do not code.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
Not one single person is gonna read all that
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Can you comment
on TOS code quality
Any weird
design choices Terry made, what he does well, tradeoffs, what could be better
given modern
dev practices
Jump in the discussion.
No email address required.
It's quite well written if you ignore the fact that HolyC code doesn't have scopes (oh right this is very important i forgot to add it in the post but whatever) like
so to naturally avoid weirdness all variable declaration goes on the top of the function, like old school C.
Honestly I'm not one to judge his code since im still a novice by programming standards (~1yr of experience overall) but I'd say the lack of SSE floats and using the FPU is kind of a design flaw, since it's much slower. I think he was going to add it but he died before that
Terry also mixes up the APIC, PIC and PCI in a weird way (thats why it wont boot on even remotely recent bare metal hardware) but that's too complicated to explain. Not blaming him though, schizophrenia is tough.
I found a small bug in the compiler that deals with register variables and inlinr assembly, but that's for another blogpost.
Terry does manual struct packing very well, and ive rarely ever seen any C programmer doing that despite it being a major size optimization point in many cases.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
this is not a weekend project, it took me months and i basically learned everything from zero while doing this. you can do it too friend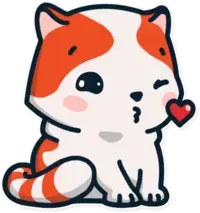
Jump in the discussion.
No email address required.
You've brightened our day by showing what dedication can do
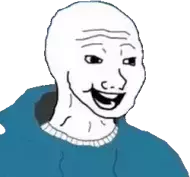
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
On the one hand: incredibly based. On the other: what the frick?
Why use such a shallow embedding? For instance, why not just source-to-source compile the HolyC to C++ and recompile the thing yourself? That seems orders of magnitude easier then getting all his asm to run natively. Is there enough that's handwritten to make that profitable?
Jump in the discussion.
No email address required.
because its TempleOS, we need the GUI. also need to keep the compoler orthodox
Jump in the discussion.
No email address required.
Sure, but you could just translate the graphics calls to SDL, which out seems like OP did anyways
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
Everyone will eventually find themselves staring down a train.
Jump in the discussion.
No email address required.
More options
Context
Very impressive!
I didn't even know HolyC was an actual thing.
How long did this take you?
Jump in the discussion.
No email address required.
thanks!
around 5 months, probably more because i started from when i basically didnt know anything
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Very interesting post!
Jump in the discussion.
No email address required.
More options
Context
I don't understand most of the things you have said here, but I know that God has found himself a new agent, and Terry can rest in peace.
Jump in the discussion.
No email address required.
More options
Context
Can't wait to be gooming the fetch Christian games he made for it.
Jump in the discussion.
No email address required.
More options
Context
Are you the genius
that did this? Or are you blog posting
about someone else?
Jump in the discussion.
No email address required.
i made it ofc, and anyone technically sophisticated enough would see this whole longpost couldnt randomly be written by a 3rd party.
but for extra confirmation i could make a secret gist saying hello rdrama or something when i get back home tomorrow
Jump in the discussion.
No email address required.
Ily
Jump in the discussion.
No email address required.
zoz
Jump in the discussion.
No email address required.
zle
Jump in the discussion.
No email address required.
zozzle
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
Jump in the discussion.
No email address required.
More options
Context
This is insanely cool even though I barely understand it once you start bringing up stack pointers and registers. Where'd you learn all this?
Jump in the discussion.
No email address required.
More options
Context