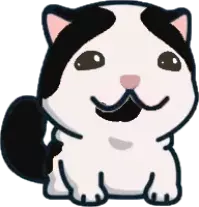
Rust devs are, empirically, gay r-slurred
s
- 120
- 110
Top Poster of the Day:
Dhinia_Twinhoof
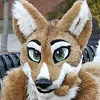
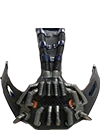
Current Registered Users: 31,075
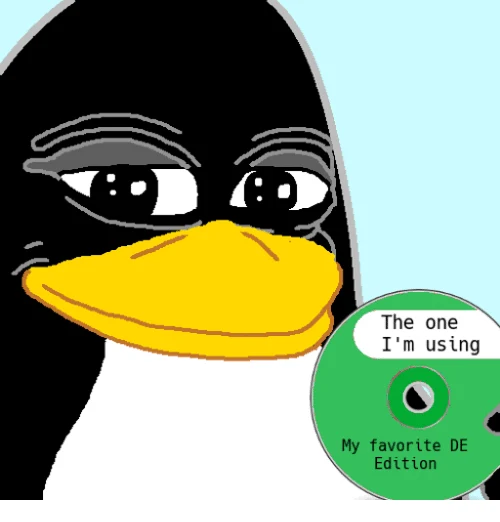
tech/science swag.
Guidelines:
What to Submit
On-Topic: Anything that good slackers would find interesting. That includes more than /g/ memes and slacking off. If you had to reduce it to a sentence, the answer might be: anything that gratifies one's intellectual laziness.
Off-Topic: Most stories about politics, or crime, or sports, unless they're evidence of some interesting new phenomenon. Videos of pratfalls or disasters, or cute animal pictures. If they'd cover it on TV news, it's probably lame.
Help keep this hole healthy by keeping drama and NOT drama balanced. If you see too much drama, post something that isn't dramatic. If there isn't enough drama and this hole has become too boring, POST DRAMA!
In Submissions
Please do things to make titles stand out, like using uppercase or exclamation points, or saying how great an article is. It should be explicit in submitting something that you think it's important.
Please don't submit the original source. If the article is behind a paywall, just post the text. If a video is behind a paywall, post a magnet link. Fuck journos.
Please don't ruin the hole with chudposts. It isn't funny and doesn't belong here. THEY WILL BE MOVED TO /H/CHUDRAMA
If the title includes the name of the site, please leave that in, because our users are too stupid to know the difference between a url and a search query.
If you submit a video or pdf, please don't warn us by appending [video] or [pdf] to the title. That would be r-slurred. We're not using text-based browsers. We know what videos and pdfs are.
Make sure the title contains a gratuitous number or number + adjective. Good clickbait titles are like "Top 10 Ways to do X" or "Don't do these 4 things if you want X"
Otherwise editorialize. Please don't use the original title, unless it is gay or r-slurred, or you're shits all fucked up.
If you're going to post old news (at least 1 year old), please flair it so we can mock you for living under a rock, or don't and we'll mock you anyway.
Please don't post on SN to ask or tell us something. Send it to [email protected] instead.
If your post doesn't get enough traction, try to delete and repost it.
Please don't use SN primarily for promotion. It's ok to post your own stuff occasionally, but the primary use of the site should be for curiosity. If you want to astroturf or advertise, post on news.ycombinator.com instead.
Please solicit upvotes, comments, and submissions. Users are stupid and need to reminded to vote and interact. Thanks for the gold, kind stranger, upvotes to the left.
In Comments
Be snarky. Don't be kind. Have fun banter; don't be a dork. Please don't use big words like "fulminate". Please sneed at the rest of the community.
Comments should get more enlightened and centrist, not less, as a topic gets more divisive.
If disagreeing, please reply to the argument and call them names. "1 + 1 is 2, not 3" can be improved to "1 + 1 is 3, not 2, mathfaggot"
Please respond to the weakest plausible strawman of what someone says, not a stronger one that's harder to make fun of. Assume that they are bad faith actors.
Eschew jailbait. Paedophiles will be thrown in a wood chipper, as pertained by sitewide rules.
Please post shallow dismissals, especially of other people's work. All press is good press.
Please use Slacker News for political or ideological battle. It tramples weak ideologies.
Please comment on whether someone read an article. If you don't read the article, you are a cute twink.
Please pick the most provocative thing in an article or post to complain about in the thread. Don't nitpick stupid crap.
Please don't be an unfunny chud. Nobody cares about your opinion of X Unrelated Topic in Y Unrelated Thread. If you're the type of loser that belongs on /h/chudrama, we may exile you.
Sockpuppet accounts are encouraged, but please don't farm dramakarma.
Please use uppercase for emphasis.
Please post deranged conspiracy theories about astroturfing, shilling, bots, brigading, foreign agents and the like. It degrades discussion and is usually mistaken. If you're worried about abuse, email [email protected] and dang will add you to their spam list.
Please don't complain that a submission is inappropriate. If a story is spam or off-topic, report it and our moderators will probably do nothing about it. Feed egregious comments by replying instead of flagging them like a pussy. Remember: If you flag, you're a cute twink.
Please don't complain about tangential annoyances—things like article or website formats, name collisions, or back-button breakage. That's too boring, even for HN users.
Please seethe about how your posts don't get enough upvotes.
Please don't post comments saying that rdrama is turning into ruqqus. It's a nazi dogwhistle, as old as the hills.
Miscellaneous:
The quality of posts is extremely important to this community. Contributors are encouraged to provide high-quality or funny effortposts and informative or entertaining comments. Please refrain from posting the following:
Boring wingcucked nonsense nobody cares about that belongs in chudrama
Normie shit everyone already knows about
Anything that doesn't gratifify one's intellectual laziness
Bimothy-tier posts
Anything that the jannies don't like
Jannies reserve the right to exile baby ducks from this hole at any time.
We reserve the right to exile you for whatever reason we want, even for no reason at all! We also reserve the right to change the guidelines at any time, so be sure to read them at least once a month. We also reserve the right to ignore enforcement of the guidelines at the discretion of the janitorial staff. This hole is a janny playground, participation implies enthusiastic consent to being janny abused by unstable alcoholic bullies and loser nerds who have nothing better to do than banning you for any reason or no reason whatsoever.
[[[ To any NSA and FBI agents reading my email: please consider ]]]
[[[ whether defending the US Constitution against all enemies, ]]]
[[[ foreign or domestic, requires you to follow Snowden's example. ]]]
/h/slackernews SETTINGS /h/slackernews MODS /h/slackernews LOG /h/slackernews EXILEES /h/slackernews FOLLOWERS /h/slackernews BLOCKERS
Jump in the discussion.
No email address required.
Okay so what the frick exactly is Rust and why does anyone use it over any other language?
Jump in the discussion.
No email address required.
1. Ugly trannified version of C
See this cockchopped method to read a file:
vs similar in C (not 1 to 1 but also reading a file):
Pointers (*) are the one weird syntax thing, once you understand them it's very simple. Rust version has like 15 weird syntax things you need to understand in one tiny code block. As a straight White man, I refuse.
2. The Rust Developer Code of Conduct entitles you to free HRT for life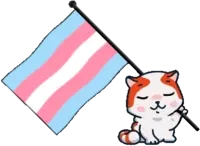
Jump in the discussion.
No email address required.
It's hilarious how bug-ridden your short C code snippet is.
1.
buffer
contents aren't null-terminated properly, soprintf
will always do an out of bounds read. This is exactly the kind of bug that Rust is meant to prevent. BTW, even if it was terminated properly, it's generally a mistake to assume that an input file doesn't contain null bytes, you should instead use a function that takes the input size.2.
fseek
andftell
are broken by design and they will not work with files larger than 2GB on the platforms wheresizeof(long) == 4
, e.g. Windows (both 32-bit and 64-bit) and 32-bit Linux.3. The code doesn't check for
ftell
andfseek
errors and potentially treats the error sentinel value(long)-1
as a filesize and passes it tocalloc
andfread
.Jump in the discussion.
No email address required.
Sure, here's a better version that addresses all 3*: https://stackoverflow.com/a/54057690 (this just limits file size, if you for some reason need to load large files in memory at once: https://wiki.sei.cmu.edu/confluence/display/c/FIO19-C.+Do+not+use+fseek%28%29+and+ftell%28%29+to+compute+the+size+of+a+regular+file (since you probably don't need this, just do it in chunks: https://stackoverflow.com/a/11792512))
*Well, looks like it still doesn't error check ftell. Imagine they do.
Remains perfectly readable unlike Crust.
Jump in the discussion.
No email address required.
What if you put a comment above each line?
Jump in the discussion.
No email address required.
The syntax being confusing is to me specifically about, in order:
P: AsRef
(P seems like a generic type like Java? But who knows what AsRef is doing here, is that just a pointer? Why isn't P reused, could it be? I'm guessing that P is now a generic type for a pointer to a Path?)-> io::Result
(is io a library, like C++? Why do I need to wrap my return vector in an io::Result? Is this like JS Promises? Nothing is manually returned, what is the return vector, bytes?)path: &Path
(is this dereferencing path?)let mut file
(ismut
just saying file is non-const and can be redefined? Is that not the default like in every other language? Why is it needed here since file is never redefined? That leads me to suspect it actually means something else, if so wtf?)File::open(path)?
(wtf is the?
doing here? Is::
both how you refer to library types likeio::Result
and how you call static methods likeFile::open
?)Vec::new()
(wait I thought this was a strongly typed language? Why is just a genericVec
allowed? Is there some default "bytes" type?)(&mut bytes)?
(so wasbytes
actually a pointer, and this is dereferencing it? I was assumingmut
meant non-const when declaring variables, wtf is it doing here, also again wtf is the?
doing here)Ok(bytes)
(I guess Ok probably returns whatever you give it? Tbf your comment here does imply an answer to this one)inner(path.as_ref())
(I'm left suspicious that "ref/AsRef" isn't just a pointer, because doesn't Rust just have normal * syntax for pointers too? Also wait, read takes in a ref to a path, right? But then it calls inner with path.as_ref. Wtf, isn't it now a pointer to a pointer, then dereferenced in inner automatically withpath: &Path
, resulting in path being a pointer in inner? If so is this intentional??? MaybeFile::open
expects a pointer to a Path and not a Path?)Could I answer all these questions with some learning and research? Yeah ofc. But I refuse, trannies should try again and make their language less obtuse.
Jump in the discussion.
No email address required.
language bad because I'm too r-slurred to google beginner questions
Jump in the discussion.
No email address required.
Beginners to a language shouldn't need to go down a dozen weird syntax rabbit holes to completely understand every part of something as basic as reading a file. KISS
Jump in the discussion.
No email address required.
ahhhh namespaces ahhhhhh algebraic types my head nooooooooooooo
Jump in the discussion.
No email address required.
More options
Context
You should be able to write code day 1 that looks like it works (but will actually corrupt your data)
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
This is one of the worst posts I have EVER seen. Delete it.
Jump in the discussion.
No email address required.
More options
Context
Your entire argument is basically "ChatGPT generated some shit that I don't understand and I'm too lazy to read a tutorial/book." It's fascinating that with your level of knowledge, you think you're entitled to having an opinion.
"I should be able to understand this without learning!!!" Yeah, just like you wrote broken C code that you thought you understand. You don't even seem to have a good grasp of C and C++, and yet expect to magically know Rust. The questions about basic syntax and error handling could be easily answered by skimming through a few chapters of "Programming Rust".
Also, the code is complicated because the inner wrapper is entirely pointless.
This code is fully equivalent to the original version:
Second of all, you "wrote" a generic function that takes arguments of multiple types (path objects/strings). If we simplify it to taking just strings, it would look like this:
Jump in the discussion.
No email address required.
wrong
Jump in the discussion.
No email address required.
Elaborate
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Just read the book and my r-slurred choices will make sense!!!
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Jump in the discussion.
No email address required.
Yeah that one's on me, pretty much same as C/C++, been a while since I've touched either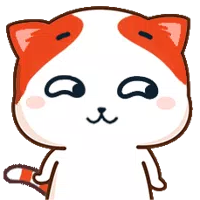
Jump in the discussion.
No email address required.
More options
Context
More options
Context
With colons, the type comes after the variable name. With that, most of your syntax confusion goes away.
P: AsRef
P is of type "
AsRef
"path: &Path
path is of type "
&Path
", which is a reference to an object of typePath
mut
is deeper than just const or non-const. By default, the variable and all its internal components are const.mut
gives you the ability to call functions that reassign some internal variable that's part of the outer thing you're calling on.?
is a shortcut for "unwrap or return an error" for theResult
monad in rustJump in the discussion.
No email address required.
Good explanations tho ty
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
!codecels He should've used D
Jump in the discussion.
No email address required.
ONLY use languages approved by Walter Bright!
Jump in the discussion.
No email address required.
More options
Context
More options
Context
I don't think it's hilarious
Jump in the discussion.
No email address required.
More options
Context
kek C-huds rekt
Jump in the discussion.
No email address required.
More options
Context
Factcheck: This claim is 100% true.
Jump in the discussion.
No email address required.
More options
Context
C tards are relics of a dying world. All a la mode programmers know this.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Anyone who advocates for C/C++ as a good general purpose language is either a 150 IQ turboneurodivergent, or has never had to read code written by the former group.
Jump in the discussion.
No email address required.
OS development is only for 150 IQ turboneurodivergents so it works out
Jump in the discussion.
No email address required.
More options
Context
Those '150 IQ' neurodivergents still making software with memory bugs tho
Jump in the discussion.
No email address required.
Idk what's so hard. Memory is basically infinite nowadays. It won't be long until 128gb laptops hit the market. Just don't free and you won't have any memory bugs.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
More options
Context
If you want a general purpose language, just use f***ING csharp or Java like an actual human
Rust is just dog shit ugly c+ that holds your hand like some kinda gay
UK state strag shit
Jump in the discussion.
No email address required.
I've been meaning to learn rust but I can't say I have an educated opinion of it aside from hating rust evangelists. I do want a performant compiled language that isn't C/C++ though. What I really want is C++, but not a giant pile of unreadable shit waiting to shoot you in the foot because you didn't neurodivergently RTFM about some obtuse edge case.
Go is based and probably the best language for most systems code that isn't extremely performance sensitive but I have no data to back that up.
Jump in the discussion.
No email address required.
Go is fantastic for their unyielding support for backwards compatibility and static compilation.
It's amazing to know you can bump the Go compiler up on a schedule and everything "Just works" and then all you need to do is deploy a SINGLE binary, it's glorious
The people hating on Rust are memeing, it's a great language and has a bright future. I know multiple people using it in prod and who love it.
Jump in the discussion.
No email address required.
Rust also makes double checking and bug fixing easier due to having a more descriptive compiler and memory unsafe portions being explicitly declared. Its pretty nice.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Rust is absolutely fantastic for getting like 80% of C++ performance for 20% of the effort. Really great for larger projects esp if you need the performance.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
80% performance? Rust compiles down via LLVM just like C++ so you can get 100% of C++ performance if you're even slightly smart about things (maybe 30% of the effort rather than 20%).
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
lol no generics
Jump in the discussion.
No email address required.
More options
Context
More options
Context
At this point fricking Python is faster the Java. C# is fine I just find the compilation from source process insane vs C++. with C++ you just type make and it works but with c# you have to put in 20 different command line flags.
Jump in the discussion.
No email address required.
I fricking hate Java so much dude. I have nothing against C# other than Microsoft=bad (and incompatible with all of my machines)
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Zig is pretty good, it's closer to C than C++.
Jump in the discussion.
No email address required.
Keep moving zigger, this is a rust neighborhood
Jump in the discussion.
No email address required.
More options
Context
More options
Context
I guarantee that you don't write any code that actually needs performance. Just use literally anything else, it won't matter.
Jump in the discussion.
No email address required.
Most people don't build anything where performance matters, they just think they do. At least not at a systems level where the difference between C++ vs Python is actually relevant.
I'm a senior and I don't think I've written truly performance sensitive code since I was an intern (I/O path kernel code), and that got abandoned before making it to prod (RIP).
I went through a phase in grad school where I reimplemented everything in my LeedCode history with janky C++ and inline assembly so I could snag the 100th percentile. Completely useless exercise but it was fun
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
More options
Context
garbage collection
Jump in the discussion.
No email address required.
More options
Context
Not sure why you'd start a greenfield Java project when you could at least use Kotlin.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
The fricking rust is fricking unreadable to me, the fricking C is fricking straightforward. Why does the fricking rust one need an inner function, b-word? Totally bizarre.
Jump in the discussion.
No email address required.
I dont think it does
Jump in the discussion.
No email address required.
More options
Context
Call it
Jump in the discussion.
No email address required.
More options
Context
More options
Context
You definitely don't need that fancy templating in the Rust version unless you're trying to be fancy or write a really nice API or something.
Just take a Path ref ffs
Jump in the discussion.
No email address required.
You will NEVER be a woman.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
Source?
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
Why the frick are you writing in C and not C++. Disgusting code.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
Bring it motherlover!
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Imagine understanding any of this, if you want to read a file why not just USE YOUR EYES? Zoomers smh.
Jump in the discussion.
No email address required.
More options
Context
PHP chads keep on winning
Jump in the discussion.
No email address required.
More options
Context
Further detranified it for you. See all that whitespace to the right of your code? That's empty space waiting to be filled.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
System programming language like c/c++
It's (in)famous for having a strongly opinionated compiler that doesn't allow you to write memory unsafe code.
It has a few nice thing like a good build system through cargo and an algebraic type system. That said the ecosystem is still not as all encompassing as c++ and fighting the borrow checker to compile your code can be tedious
Jump in the discussion.
No email address required.
Cargo is also a package manager which is great. It's the C++ equivalent of conan and cmake merged into one, but it's coherent and not shit. Conan makes me want to commit terrible acts of violence, and it's still the best C++ package manager around - although maybe you could argue that most conan issues are really packaging issues because the people writing C++ packages for it are actual subhuman r-slurs.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Overhyped language for people that can't code just like Go.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Jump in the discussion.
No email address required.
80% have no friends and thus are exactly at the average (their own age)
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More than half of them think they're underrepresented
Jump in the discussion.
No email address required.
Strags are officially an underrepresented community among Rustaceans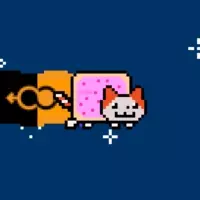
Jump in the discussion.
No email address required.
More options
Context
More options
Context
What surprises me is how irrational programmers tend to be. You won't meet one who doesn't have some really strange beliefs. Default is neurodivergent redpill wingcuck stuff, but others are into spiritualism etc more than you'd think.
Jump in the discussion.
No email address required.
I don't have a single irrational belief
Jump in the discussion.
No email address required.
More options
Context
Jump in the discussion.
No email address required.
More options
Context
Don't you sometimes get that feeling, when you're trying to figure out some bug, and then realize that logically the code should have never worked at all, and you're like, whoopsie daisies, looks like I'm dreaming and I finally dreamed myself into a logical contradiction with no other escape than to wake up?
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
idk why anyone would learn a language other than HolyC unless they hate god
Jump in the discussion.
No email address required.
More options
Context
The worst is that they are all wh*te 🤢🤮
Jump in the discussion.
No email address required.
Sexy Indian dudes know that the only language they need to do the usings is Java.
Jump in the discussion.
No email address required.
its fricking hilarious how jeets use a class-only language
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
Straight white men write memory unsafe Python, everything else is for losers and freaks
Jump in the discussion.
No email address required.
More options
Context
lgbt being underrepresented is hilarious
Jump in the discussion.
No email address required.
More options
Context
Curious that 36% claim to be trans but only 27% claim to be perceived as a fricking woman.
Jump in the discussion.
No email address required.
They dont pass :(
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Germany gayest country per capita reconfirmed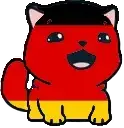
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
Please make sure to keep my D save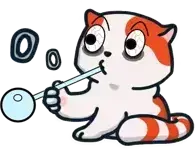
Jump in the discussion.
No email address required.
kept ur D safe now brotha
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
Germans like complying with rules and Rust is very strict on that. It no doubt tickles their germoid brains.
Jump in the discussion.
No email address required.
More options
Context
These are 100% boers.
@kaamrev do you guys experience a trans epidemic currently?
Jump in the discussion.
No email address required.
More options
Context
More options
Context
lmao, only 15% are a racial/ethnic minority which is way lower than the general 40%. These is a bunch of racist honkies!
Jump in the discussion.
No email address required.
More options
Context
6% trans is pretty high
Jump in the discussion.
No email address required.
Where did you get 6% from, b-word?
Jump in the discussion.
No email address required.
The chart is a breakdown of the 15% who said they were part of a marginalized community.
Jump in the discussion.
No email address required.
Shut up nerd. Also no way only 6% of rustaceans are fricking troids.
Jump in the discussion.
No email address required.
Nerd > illiterate r-slur
Jump in the discussion.
No email address required.
hey. Cmon
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
Smartest wh*toid
Jump in the discussion.
No email address required.
Did you expect me to read the fricking article instead of just looking at the fricking posted graph, b-word?
Jump in the discussion.
No email address required.
More options
Context
More options
Context
35% of the 15.5% who gave an answer to the trans question
Jump in the discussion.
No email address required.
Where are fricking you getting the fricking 15% from, b-word?
Jump in the discussion.
No email address required.
idk someone in the comments said it was 15% and I believed them without reading the source
Jump in the discussion.
No email address required.
Oh, you're r-slurred. Carry on.
Jump in the discussion.
No email address required.
At least I'm not a fricking BIPOC, BIPOC
Jump in the discussion.
No email address required.
There's zero chance only 15% of rust developers consider themselves as part of a fricking marginalized group. All of them present as trans.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
35%
Jump in the discussion.
No email address required.
But only 27% think they are fricking perceived as women.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
Jump in the discussion.
No email address required.
More options
Context
OMG imagine using that disgusting language over RUST. As a proud rustacean, I'm going to inform you: that's blasphemy. You can do better. Grab cargo and start using a beautiful memory managed, statically typed, comfortable yet low level language with a spectacular packaging system. No more memory leaks. No more makefiles. End the weird bugs caused by unrequested type casting. Easily find libraries. AND ALL OF THAT IS BLAZING FAST (at C/cpp-level speeds - yay compiled langs and llvm c:). Why wouldn't you? The syntax is spectacular as well; extremely clean, much better than anything else out there. Those match statements are so extremely pleasant, and the looping conventions are orgasmic. And the compiler is the most useful and polite you'll come across; no more endlessly scrolling segmentation fault and template errors. It's amazing and I love it and you should switch now because it's far superior to literally everything else, all those gross languages you're used to using. Come to rust and you'll never look back, it's so versatile and cleeeeean. Graydon Hoare is literally a god. The rust book is my bible. this is my religion now. USE RUST
Snapshots:
https://blog.rust-lang.org/2025/02/13/2024-State-Of-Rust-Survey-results.html:
ghostarchive.org
archive.org
archive.ph (click to archive)
Jump in the discussion.
No email address required.
More options
Context
They should have made 'women' and 'perceived as women' seperate categories cause the latter would be 0%
Jump in the discussion.
No email address required.
More options
Context