Post solutions here
Top Poster of the Day:
911roofer
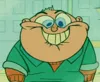
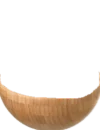
Current Registered Users: 30,843
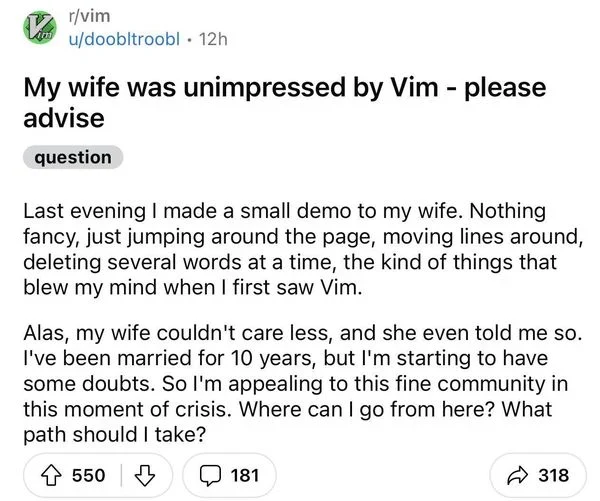
tech/science swag.
Guidelines:
What to Submit
On-Topic: Anything that good slackers would find interesting. That includes more than /g/ memes and slacking off. If you had to reduce it to a sentence, the answer might be: anything that gratifies one's intellectual laziness.
Off-Topic: Most stories about politics, or crime, or sports, unless they're evidence of some interesting new phenomenon. Videos of pratfalls or disasters, or cute animal pictures. If they'd cover it on TV news, it's probably lame.
Help keep this hole healthy by keeping drama and NOT drama balanced. If you see too much drama, post something that isn't dramatic. If there isn't enough drama and this hole has become too boring, POST DRAMA!
In Submissions
Please do things to make titles stand out, like using uppercase or exclamation points, or saying how great an article is. It should be explicit in submitting something that you think it's important.
Please don't submit the original source. If the article is behind a paywall, just post the text. If a video is behind a paywall, post a magnet link. Fuck journos.
Please don't ruin the hole with chudposts. It isn't funny and doesn't belong here. THEY WILL BE MOVED TO /H/CHUDRAMA
If the title includes the name of the site, please leave that in, because our users are too stupid to know the difference between a url and a search query.
If you submit a video or pdf, please don't warn us by appending [video] or [pdf] to the title. That would be r-slurred. We're not using text-based browsers. We know what videos and pdfs are.
Make sure the title contains a gratuitous number or number + adjective. Good clickbait titles are like "Top 10 Ways to do X" or "Don't do these 4 things if you want X"
Otherwise editorialize. Please don't use the original title, unless it is gay or r-slurred, or you're shits all fucked up.
If you're going to post old news (at least 1 year old), please flair it so we can mock you for living under a rock, or don't and we'll mock you anyway.
Please don't post on SN to ask or tell us something. Send it to [email protected] instead.
If your post doesn't get enough traction, try to delete and repost it.
Please don't use SN primarily for promotion. It's ok to post your own stuff occasionally, but the primary use of the site should be for curiosity. If you want to astroturf or advertise, post on news.ycombinator.com instead.
Please solicit upvotes, comments, and submissions. Users are stupid and need to reminded to vote and interact. Thanks for the gold, kind stranger, upvotes to the left.
In Comments
Be snarky. Don't be kind. Have fun banter; don't be a dork. Please don't use big words like "fulminate". Please sneed at the rest of the community.
Comments should get more enlightened and centrist, not less, as a topic gets more divisive.
If disagreeing, please reply to the argument and call them names. "1 + 1 is 2, not 3" can be improved to "1 + 1 is 3, not 2, mathfaggot"
Please respond to the weakest plausible strawman of what someone says, not a stronger one that's harder to make fun of. Assume that they are bad faith actors.
Eschew jailbait. Paedophiles will be thrown in a wood chipper, as pertained by sitewide rules.
Please post shallow dismissals, especially of other people's work. All press is good press.
Please use Slacker News for political or ideological battle. It tramples weak ideologies.
Please comment on whether someone read an article. If you don't read the article, you are a cute twink.
Please pick the most provocative thing in an article or post to complain about in the thread. Don't nitpick stupid crap.
Please don't be an unfunny chud. Nobody cares about your opinion of X Unrelated Topic in Y Unrelated Thread. If you're the type of loser that belongs on /h/chudrama, we may exile you.
Sockpuppet accounts are encouraged, but please don't farm dramakarma.
Please use uppercase for emphasis.
Please post deranged conspiracy theories about astroturfing, shilling, bots, brigading, foreign agents and the like. It degrades discussion and is usually mistaken. If you're worried about abuse, email [email protected] and dang will add you to their spam list.
Please don't complain that a submission is inappropriate. If a story is spam or off-topic, report it and our moderators will probably do nothing about it. Feed egregious comments by replying instead of flagging them like a pussy. Remember: If you flag, you're a cute twink.
Please don't complain about tangential annoyances—things like article or website formats, name collisions, or back-button breakage. That's too boring, even for HN users.
Please seethe about how your posts don't get enough upvotes.
Please don't post comments saying that rdrama is turning into ruqqus. It's a nazi dogwhistle, as old as the hills.
Miscellaneous:
The quality of posts is extremely important to this community. Contributors are encouraged to provide high-quality or funny effortposts and informative or entertaining comments. Please refrain from posting the following:
Boring wingcucked nonsense nobody cares about that belongs in chudrama
Normie shit everyone already knows about
Anything that doesn't gratifify one's intellectual laziness
Bimothy-tier posts
Anything that the jannies don't like
Jannies reserve the right to exile baby ducks from this hole at any time.
We reserve the right to exile you for whatever reason we want, even for no reason at all! We also reserve the right to change the guidelines at any time, so be sure to read them at least once a month. We also reserve the right to ignore enforcement of the guidelines at the discretion of the janitorial staff. This hole is a janny playground, participation implies enthusiastic consent to being janny abused by unstable alcoholic bullies and loser nerds who have nothing better to do than banning you for any reason or no reason whatsoever.
[[[ To any NSA and FBI agents reading my email: please consider ]]]
[[[ whether defending the US Constitution against all enemies, ]]]
[[[ foreign or domestic, requires you to follow Snowden's example. ]]]
/h/slackernews SETTINGS /h/slackernews MODS /h/slackernews LOG /h/slackernews EXILEES /h/slackernews FOLLOWERS /h/slackernews BLOCKERS
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
Impressive. Normally people with such severe developmental disabilities struggle to write much more than a sentence or two. He really has exceded our expectations for the writing portion. Sadly the coherency of his writing, along with his abilities in the social skills and reading portions, are far behind his peers with similar disabilities.
Jump in the discussion.
No email address required.
More options
Context
Jump in the discussion.
No email address required.
i didn't say it was good
code
also yes my code
getting marsified is pretty
funny
imo
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
this one was crazy, mostly because I kept misunderstanding the problem and making really pretty but incorrect solutions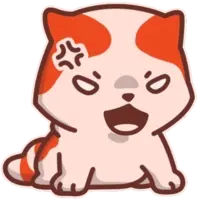
Jump in the discussion.
No email address required.
Good job friend
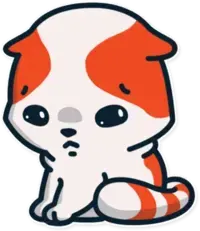
Jump in the discussion.
No email address required.
More options
Context
More options
Context
I think I'm about done with this. I've solved it but somewhere I must have gotten an array index off by one. I can't get a Python IDE running right now (long story) so it would take forever to find my mistake. And I can't really justify doing something that tedious when I won't learn anything from it.
Does it get any more interesting in later weeks or is it just gonna be more parsing text files and lists of lists? I may not be neurodivergent enough for this.
Jump in the discussion.
No email address required.
You can try to learn how to write it in a way that makes off by 1 errors significantly less likely.
Also, use print statements
, and don't neglect testing it on example input.
It's going to require somewhat nontrivial algorithms sometimes (even today, if the input was a couple of times larger, you'd probably had to solve part2 more efficiently than looking from each tree), but also yeah, expect tasks that, if you don't consciously and purposefully seek to eliminate tedium, can easily overwhelm you with it.
Jump in the discussion.
No email address required.
Well if I wanted to do that, I just wouldn't use Python.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
You can just change the url to 2021 and see what it turns into for each day. Decide how painful it looks
Jump in the discussion.
No email address required.
Randomly choose 2021 day 22 and it's just this but 3-d with a touch of combinatorics.
Jump in the discussion.
No email address required.
Day 23 looked kinda fricked tbh
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
unironically, you are learning as much from finding the off-by-one error as you are from earlier AoCs. getting complex things right like that and understanding why is critical in being a good coder.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
got tripped up for a long time by the dumbest stuff on this one. for the first part i was giving tlm() the wrong slices (row[i+1:] instead of row[x+1:] etc) then in the second part i was reversing the wrong slices, which annoyingly gave me the right answer for the example input
Jump in the discussion.
No email address required.
first i was fricking up column definitions when looping, which made me think i was fricking up slicing. then i was fricking up view distances by feeding the wrong arrays into the wrong variables. then i was fricking up view distances both in making infinite loops and in appropriately scoring views.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
Sorry ma'am, looks like his delusions have gotten worse. We'll have to admit him.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
I shouldn't share this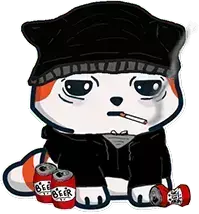
Jump in the discussion.
No email address required.
It looks pretty good to me
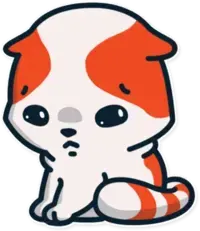
Jump in the discussion.
No email address required.
More options
Context
More options
Context
You can make things somewhat easier for yourself by using 2d index vectors and
It didn't get rid of all the bullshit, but of enough of it imo:
Jump in the discussion.
No email address required.
this but also by making a complexity monster
. I hope the input's dimension won't get too high in the next days
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
das rite, any problems with dat, white boi?
Jump in the discussion.
No email address required.
Yes i have , Use std::array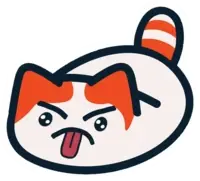
Jump in the discussion.
No email address required.
https://yosefk.com/c++fqa/picture.html#fqa-6.15
Jump in the discussion.
No email address required.
Coming from the guy using iostream
https://yosefk.com/c++fqa/io.html#fqa-15.1
Jump in the discussion.
No email address required.
Yes.
the FQA is correct, that doesn't meant that std::istream & co aren't convenient for basic tasks
Jump in the discussion.
No email address required.
true king , let's hope "std::print" will in the future replace "ostream" though.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
you can tell how smart someone is from their solution lol, makes sense you're from motte
an even better solution is just numpy tho:
Jump in the discussion.
No email address required.
More options
Context
Jesse what the frick are you talking about??
Jump in the discussion.
No email address required.
More options
Context
Jump in the discussion.
No email address required.
More options
Context
More options
Context
I'm sure there are neat ways to iterate through rotations, but fuck it, brute force gang 4 lyffixed lolJump in the discussion.
No email address required.
What language is this?
Jump in the discussion.
No email address required.
APL
the old one
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
i cri
Jump in the discussion.
No email address required.
More options
Context
Jump in the discussion.
No email address required.
More options
Context
Almost got both of them done as 1-liners, I could do it if I remembered how to use Haskell.
Jump in the discussion.
No email address required.
now what's the big O of this mess?
Jump in the discussion.
No email address required.
About as big as your mom.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
Jump in the discussion.
No email address required.
More options
Context
python would be much better for this bc slices and sum()/max() or just numpy
Jump in the discussion.
No email address required.
Every time I read one of your answers I feel like you're one of those people who intentionally makes their code illegible so that they keep you around just so nobody else has to go figure out what you did.
Jump in the discussion.
No email address required.
the above is much simpler than the other solutions though. the names are shit because it's just a game but 'viewacc' takes a function and applies it to each tree's list of adjacent trees going out north, south, east, and west. so you pass it a function that takes the tree's value, and then four lists of tree values. this lets you make the two problems easier to understand, and the code for them doesn't have to worry about anything other than those four lists, and can treat each direction the same way. it's pre messy because vanilla js + written quickly, the numpy solution is much smaller and makes more sense, but if things were given better names it'd be much better than the others.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
There's probably some way to simplify the visibility search instead of a separate for loop for each direction. I'm too lazy to find it
Jump in the discussion.
No email address required.
If only you could put that energy into your relationships
Jump in the discussion.
No email address required.
More options
Context
More options
Context
With the magic of transpose
it still took me too long. Cleaned up my mess
Jump in the discussion.
No email address required.
More options
Context
done, had a high star delta compared to how it should've been since I did the part 1 in reverse, as in I did it from the edges and not from every element. I saw some optimisations for p2 but I'm too lazy to do it.
matlab (
), posting forest pics next post
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Not very pretty, but I was able to write it quickly
I had to fix a couple of off-by-one errors, and thought it would hurt my score more than it did
Jump in the discussion.
No email address required.
If only you could put that energy into your relationships
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Anyone come up with an algorithm for part 2? For part 1, I created a map of the maximum value from each direction at any given point, which reduced the iterations to 4x the total number of trees, but for part 2 I just brute forced it by looking from every single tree. It would be interesting to see if there was, for example, some way of determining the left viewing distance during a single pass through a line from left to right.
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
c++
part two took me way to long to figure out, how to consider the difference between a tree being on the edge and seeing 0 and a tree only seeing its neigbor.
Jump in the discussion.
No email address required.
Wtf does the parser do for this to happen
???
Jump in the discussion.
No email address required.
aevann doing the advent of fixing code blocks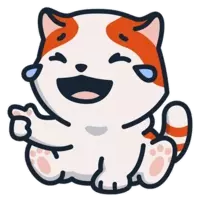
Jump in the discussion.
No email address required.
yep
Jump in the discussion.
No email address required.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
That's great and all, but I asked for my burger without cheese.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
would've been easier if i wasn't so damn dyslexic
now that i think of it, there's probably a O(n) solution for PART 2, i'm just a bit lazy on figuring it out.
Jump in the discussion.
No email address required.
Some people are able to display their intelligence by going on at length on a subject and never actually saying anything. This ability is most common in trades such as politics, public relations, and law. You have impressed me by being able to best them all, while still coming off as an absolute idiot.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Tried to do a repetitive version optimized to solve part 1 (only do a single pass of each row/col instead of calculating visibility for each coord independently), but that bit me slightly for part 2.
Jump in the discussion.
No email address required.
Posts like this is why I do Heroine.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
It's incredibly hideous, inefficient, and it took me hours to isolate the typos I had made but it's finally done just in time for Day 9
Part 1:
Part 2:
Jump in the discussion.
No email address required.
Posts like this is why I do Heroine.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Just messily brute forced this one, there's probably a far better way of doing it. Took about 20 minutes overall.
Jump in the discussion.
No email address required.
I don't have enough spoons to read this shit
Jump in the discussion.
No email address required.
More options
Context
More options
Context
You are actually too low iq to get a simple joke.....
Jump in the discussion.
No email address required.
More options
Context