I won't paste my code yet since my parser just does the needful, and I somehow manage to get overlapping grains of sand, though that doesn't matter in the end.
I'm also seeing a lot of pythonistas having high runtime (1-10s+ and more) and I'm here with the bruteforce grain-by-grain taking 150ms for part 2 (including drawing the final graph lmao). Matlab chads can't stop winning
EDIT : part 2 webm
Jump in the discussion.
No email address required.
Today's problem was fairly easy. I adopted a brute force approach since who cares, I am not gonna die for using ~2MiB of system memory, maybe it's slow but surely it works
Jump in the discussion.
No email address required.
Tbh there is a pretty easy way to optimize it. Instead of dropping it from the source, just save the full path and drop it from the last valid position, saves a lot of time.
If you just want to optimize part 2, just make a wave.
Jump in the discussion.
No email address required.
Yeah you're right. Thankfully the inputs' dimensions are not too high
Jump in the discussion.
No email address required.
Yep. Fortunately in matlab I can easily add columns so I didn't need to hardcode a big playspace, but I've seen some people get fricked because they didn't hardcode it high enough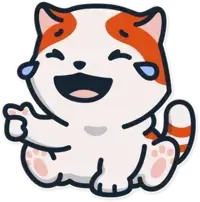
Jump in the discussion.
No email address required.
Matlabs chads simply cannot stop winning
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
More options
Context
More options
Context
little late guys:
too lazy to remove debug code
Jump in the discussion.
No email address required.
All them words won't bring your pa back.
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Harcoded the area and misread the instruction so my parttwo stops if the sand is one tile below the source. So naturally i just moved the source up by one.
Jump in the discussion.
No email address required.
look im gunna have 2 ask u 2 keep ur giant dumps in the potty not in my replys 😷😷😷
Jump in the discussion.
No email address required.
More options
Context
More options
Context
I probably overdid it since I assume there's a way of simulating it without recreating the entire temple structure in matrix format, but doing it that way seemed more fun.
Jump in the discussion.
No email address required.
More options
Context
brute force solution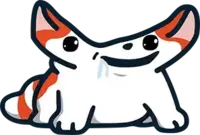
Jump in the discussion.
No email address required.
More options
Context
finally a problem where i don't have to learn some bullshit al-ghul-rhythms
Jump in the discussion.
No email address required.
More options
Context
Shit I got work today, gonna be lateeeeee
Jump in the discussion.
No email address required.
More options
Context
ogey that's moderately washed
my parser is horrible but works fine
40-50ms for the whole thing
Jump in the discussion.
No email address required.
More options
Context
Jump in the discussion.
No email address required.
MIT Scheme doesn't have this problem
Jump in the discussion.
No email address required.
More options
Context
I ran into the same thing because I was only rendering walls in one direction (that coincidentally worked for the example, just not the real data).
Jump in the discussion.
No email address required.
More options
Context
More options
Context
My code literally takes only 0.001ms
Jump in the discussion.
No email address required.
That's roughly 60,000,000 times slower than me exploding in your face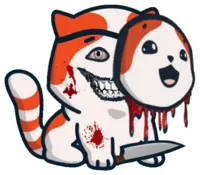
Jump in the discussion.
No email address required.
More options
Context
Let's see your program then
Jump in the discussion.
No email address required.
You wanna steal it huh
Jump in the discussion.
No email address required.
More options
Context
More options
Context
Jump in the discussion.
No email address required.
You should just give up
Jump in the discussion.
No email address required.
More options
Context
More options
Context
More options
Context
IM DELETING YOU, BROTHER!
█]]]]]]]]]]]]]]]]]] 10% complete.....
████]]]]]]]]]]]]] 35% complete.....
████████]]]]]]] 60% complete.....
████████████] 99% complete.....
ERROR! Brothers of Islam are irreplaceable I could never delete you Brother!
Send this to ten other Mujahideen who would give their lives for ﷲAllahﷲ Or never get called Brother again
If you get
0 Back: Juhanam for you
3 back: you're off the martyr list
5 back: you have pleased Allah greatly
10+ back: JANAHﷲ!ﷲ
Jump in the discussion.
No email address required.
More options
Context